注解
标记某个被注解的东西(类, 方法等),让其他程序根据这个东西上面的注解信息来执行对他的操作
元注解
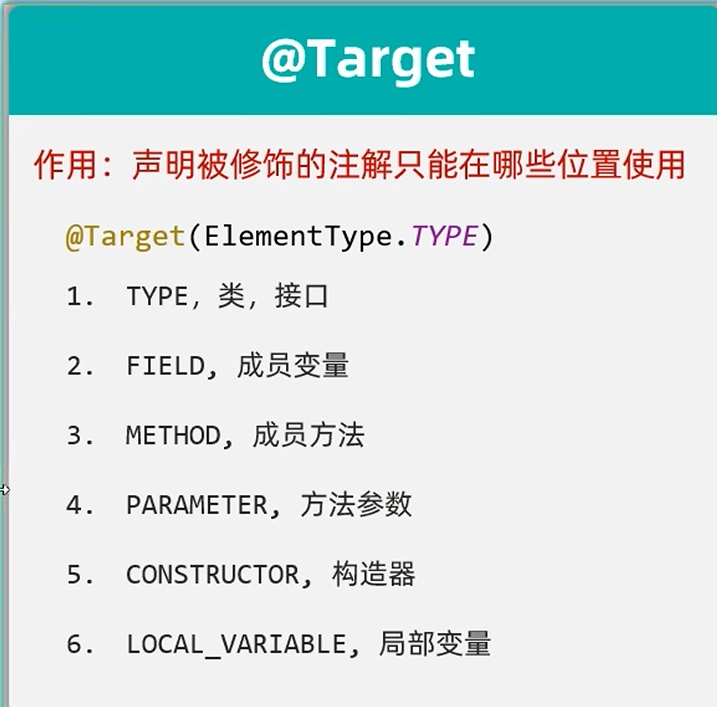
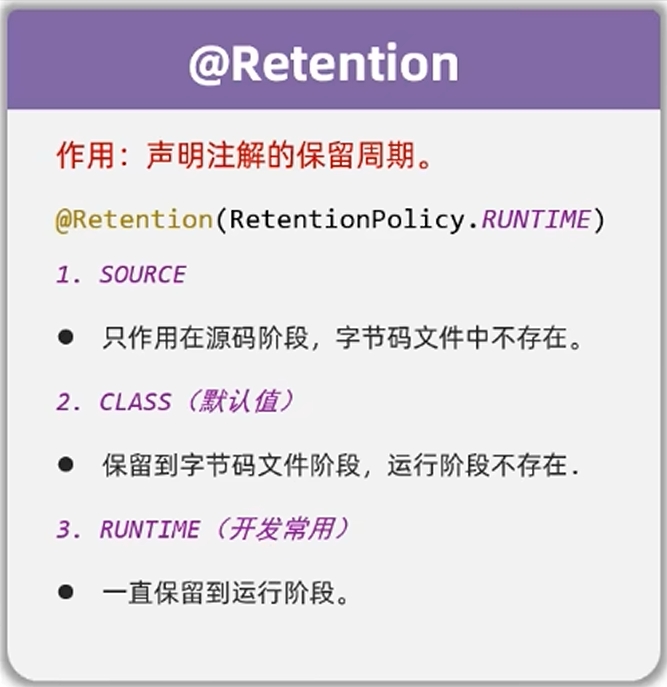
- Documented:表示注解是否被 javadoc 工具记录,默认情况下注解是不包含在 javadoc 中的。
- Inherited:表示注解是否可以被继承,默认情况下注解是不会被子类继承的。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
| @Target({ElementType.METHOD, ElementType.LOCAL_VARIABLE}) @Retention(RetentionPolicy.RUNTIME) public @interface Person { String name() default ""; int age(); }
class test {
@Person(age = 20) private static final int a = 100;
@Person(age = 18, name = "fred") public String eat(String food) { return food; } public static void main(String[] args) { try { Method eat = test.class.getMethod("eat", String.class); if (eat.isAnnotationPresent(Person.class)) { Person person = eat.getAnnotation(Person.class); String name = person.name(); int age = person.age(); System.out.println(name + " " + age); }
Field a1 = test.class.getDeclaredField("a"); Person personOnA = a1.getAnnotation(Person.class); System.out.println(personOnA.age()); } catch (NoSuchMethodException | NoSuchFieldException e) { throw new RuntimeException(e); } } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| @Retention(RetentionPolicy.RUNTIME) @Target({ElementType.METHOD}) public @interface Test { Class<? extends Throwable> expected() default None.class;
long timeout() default 0L;
public static class None extends Throwable { private static final long serialVersionUID = 1L;
private None() { } } }
|
反射
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25
| Class<?> clazz = MyClass.class;
MyClass obj = new MyClass(); Class<?> clazz = obj.getClass();
Class<?> clazz = Class.forName("com.example.MyClass");
Method[] methods = clazz.getMethods();
Method[] declaredMethods = clazz.getDeclaredMethods();
Method method = clazz.getMethod("methodName", String.class);
Field field = clazz.getDeclaredField("fieldName");
Object value = field.get(obj);
field.set(obj, "newValue");
|